Wacom one Android. Search code, repositories, users, issues, pull requests
Use saved searches to filter your results more quickly
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window. Reload to refresh your session.
The Wacom Ink SDK for devices is designed as one library. Common Device Library (CDL). that can handle different types of pen or touch input via Wacom and third-party devices.
Wacom-Developer/sdk-for-devices-Android
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Name already in use
A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?
Sign In Required
Please sign in to use Codespaces.
Launching GitHub Desktop
If nothing happens, download GitHub Desktop and try again.
Launching GitHub Desktop
If nothing happens, download GitHub Desktop and try again.
Launching Xcode
If nothing happens, download Xcode and try again.
Launching Visual Studio Code
Your codespace will open once ready.
There was a problem preparing your codespace, please try again.
Files
Failed to load latest commit information.
README.md
Wacom Ink SDK for devices. Android
The Wacom Ink SDK for devices incorporates the Common Device Library (CDL) supplied as a single library that can handle different types of pen or touch input from Wacom and third-party devices. The benefits of using the CDL include:
- Less implementation effort for developers: integration of a single library gives support for a range of input devices
- The flexibility to include support for non-Wacom devices in an application
- The freedom to take full advantage of the Wacom Ink Technologies digital ink ecosystem to provide high quality digital ink rendering without sacrificing the value of raw pen data needed in processes such as signature verification
- A generic interface relieves the application code from direct control of the connected hardware
Using the library you can:
- Search for available devices
- Connect to a device
- Receive input from a connected device in the WILL Ink format
The CDL is available for a range of development platforms
Types of supported devices include:
- Wacom PHU-111 Clipboard
- Wacom Smartpad e.g. Folio, Slate
- Wacom Intuos
- Apple Pencil
Regardless of the development platform and device type a consistent approach is provided for application development:
Use the device manager to receive a list of named devices that are available for connection
Connect to a named device and retrieve properties
Use the service callback mechanism to receive data from the connected device. Depending on the type of device, data can be in the form of realtime pen strokes, or a file transfer of a completed page.
To view operation of the CDL diagrammatically:
To illustrate the Wacom Ink SDK for devices, the samples which follow are taken from the Android version of the SDK. A similar approach is followed on all platforms where sample projects are supplied as part of the SDK.
You will need a Wacom Ink SDK for devices license, issued for your application. The easiest way to initialize your license is to add it as an asset to your application and then verify it using LicenseBootstrap.
AssetManager assetManager = getAssets; try InputStream license = assetManager.open(license.lic); LicenseBootstrap.initLicense(this.getApplicationContext, LicenseBootstrap.readFully(license)); catch (IOException | LicenseTokenException e) e.printStackTrace;
Scan for Wacom Ink Devices
In order to find the available Wacom Ink Devices start a scan. To do this create a new instance of InkDeviceScanner. It will scan for Wacom Ink Devices over bluetooth. The devices should be in pairing mode in order to be found.
- Note: To enter pairing mode on a Wacom SmartPad press and hold the button on the device for 6 seconds.
The constructor of the InkDeviceScanner takes one parameter: context.
InkDeviceScanner inkDeviceScanner = new InkDeviceScanner(this);
To start scanning for devices, call the scan method of the InkDeviceScanner. It takes a single parameter. a callback through which it reports the devices that were found.
When the scanner finds a device, it is reported as InkDevice. This object provides useful information such as the devices’s name and address.
inkDeviceScanner.scan(new InkDeviceScanner.Callback @Override public void onDeviceFound(InkDeviceInfo inkDeviceInfo) );
When scanning is complete you should stop the scanner.
After a device was found from the scanner create an instance of InkDevice. It is used to communicate with and execute commands on the Wacom Ink Device. createClient takes two parameters: context and InkDevice. InkDevice should usually be created via the dedicated InkDeviceFactory. The createClient method takes two parameters: context and inkDeviceInfo. the information which helps it create the right type of client for the Ink device and connect to it. If you have already paired with a device before there is no need to scan for it again. You can just serialize the InkDeviceInfo and reuse it to recreate the the client.
inkDevice = InkDeviceFactory.createClient(this, inkDeviceInfo);
When you have finished with the ink device, or if you need to create a new client, you should first dispose it:
Connect to Wacom Ink Device
After creating the InkDevice, you need to connect to it. The connect method takes three parameters:
- InkDeviceInfo. the information about the device (found during scan or persisted from previous usages).
- appId. a unique identifier of the application. It is used by the Ink devices to pair with the application.
- ConnectionCallback. the callback through which to be notified once the connection was successfully established.
inkDevice.connect(inkDeviceInfo, appId, new ConnectionCallback @Override public void onConnected );
There are certain things that a Wacom Ink Device can do but in most cases there are differences between one device and another. In CDL these features are grouped into sets called Device Services. To get the feature sets of the currently connected Ink device you can use the method getAvailableDeviceServices. which will return a List of DeviceServiceTypes.
ArrayListDeviceServiceType availableDeviceServices = inkDevice.getAvailableDeviceServices;
Therefore, you can easily check if the Device Service you are interested in (for example. File Transfer Service ) is available:
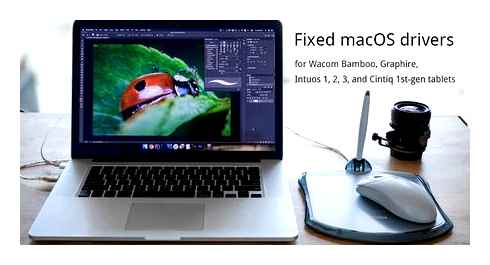
availableServices.contains(DeviceServiceType.FILE_TRANSFER_DEVICE_SERVICE)
Some of the Device Services (such as FileTransferDeviceService and LiveModeDeviceService ) cannot work in parallel. if one of them is enabled, the other cannot be started until the first one is stopped. However, Device Services like EventDeviceService can work while there is another enabled service. EventDeviceService provides the means to subscribe for and receive events sent by the device. Examples for such events are changes in the battery state, or when the device expects a certain action from the user. for example pressing the button on the Ink Device. To be able to receive these events you should first of all check if EventDeviceService is available and if so, execute its subscribe method, passing a callback through which events will be dispatched later on. There are three main types of events you will receive:
- Event. the basic event, broadcast by the Ink device (e.g. change of the battery state).
- UserActionExpected. when a user action is expected by the user.
- UserActionCompleted. tells you that the user action previously requested is now completed (either successfully or not).
if(inkDevice.getAvailableDeviceServices.contains(DeviceServiceType.EVENT_DEVICE_SERVICE)) ((EventDeviceService) inkDevice.getDeviceService(DeviceServiceType.EVENT_DEVICE_SERVICE)).subscribe(new EventCallback @Override public void onEvent(InkDeviceEvent event, Object value) @Override public void onUserActionExpected(UserAction userAction) @Override public void onUserActionCompleted(UserAction userAction, boolean success) );
Ink devices have properties which describe some of their characteristics such as name, battery state, ID and other. You can easily acquire them using the getProperties method, passing a List of the properties you are interested in. As a result, in the callback you should receive a TreeMap with property type. value pairs. The tree map keeps the same order as the list. However, if a parameter is not supported by the current Ink Device it will be omitted.
ListInkDeviceProperty properties = Arrays.asList(InkDeviceProperty.values); inkDevice.getProperties(properties, new GetPropertiesCallback @Override public void onPropertiesRetrieved(TreeMapInkDeviceProperty, Object properties) );
Alerts are unexpected events that may lead to different behavior of the InkDevice. Subscribe to them in order to be able to maintain a proper communication with the InkDevice.
inkDevice.subscribe(new AlertsCallback @Override public void onAlert(InkDeviceAlert alert) );
5.2. FileTransfer- Transfer Files From Ink Device
In this section, we want to receive and preview all the files created with the Wacom Ink Device. To do so, after making sure that the FileTransferDeviceService is supported by the current device, we should get its instance:
FileTransferDeviceService fileTransferDeviceService = (FileTransferDeviceService) inkDevice.getDeviceService(DeviceServiceType.FILE_TRANSFER_DEVICE_SERVICE);
fileTransferDeviceService.enable(new FileTransferCallback @Override public boolean onFileTransfered(InkDocument inkDocument) return true; );
through the callback, you will receive the InkDocuments containing layered Ink data.
5.3. LiveMode. Get Real Time Inking
In this section, we would like to receive the data created on the Ink Device in real time. The first thing is to make sure that this feature is supported by the device. Then we are ready to get the instance of the LiveModeDeviceService :
LiveModeDeviceService liveModeDeviceService = (LiveModeDeviceService) inkDevice.getDeviceService(DeviceServiceType.LIVE_MODE_DEVICE_SERVICE);
liveModeDeviceService.enable(new LiveModeCallback @Override public void onStrokeStart(PathChunk pathChunk) @Override public void onStrokeMove(PathChunk pathChunk) @Override public void onStrokeEnd(PathChunk pathChunk, InkStroke inkStroke) @Override public void onNewLayerCreated );
There are three callbacks through which you receive data in real time:
- onStrokeStart. A new stroke was started.
- onStrokeMove. The previously started stroke is now continued.
- onStrokeEnd. The stroke was finished. Notice that contrary to the previous two methods, this one also provides as a parameter InkStroke. containing the data describing the whole stroke.
- onNewLayerCreated. A new layer was created.
For further samples check Wacom’s Developer additional samples, see https://github.com/Wacom-Developer
For further details on using the SDK see Wacom Ink SDK for devices documentation
The API Reference is available directly in the downloaded SDK.
If you experience issues with the technology components, please see the related FAQs
For further support file a ticket in our Developer Support Portal described here: Request Support
Join our developer community:
This sample code is licensed under the MIT License
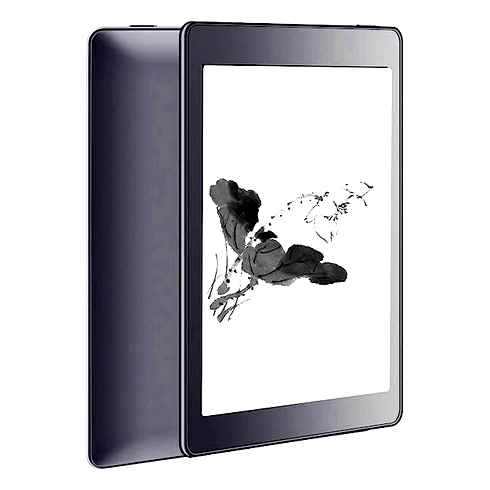
About
The Wacom Ink SDK for devices is designed as one library. Common Device Library (CDL). that can handle different types of pen or touch input via Wacom and third-party devices.
Wacom One Review
Wacom has long been the go-to brand for digital artists and creatives when it comes to pen tablets. That doesn’t mean they don’t have some serious competition, because they do. One product in particular that sees increased competition is the Wacom One Creative Pen Display tablet. This is their entry-level pen display tablet featuring a 13.3″ screen, a Wacom One pen, bundled bonus software, and compatibility with Windows, Mac, and Android. While not their top-of-the-line pen display tablet nor their best, the Wacom One is still an excellent starting point for those getting into digital media.
DESIGN
The Wacom One for the most part looks like a giant tablet, like an iPad or Android tablet, except that it doesn’t have its own OS and it’s basically just a display. The display isn’t bezel-less though and has huge ones all the way around. That’s not really a big deal though. The body is mainly made of plastic finished off in white and feels sturdy enough to last.
The back of the tablet also has two retractable risers that let you angle the tablet when in use. These are also pretty sturdy and don’t have much flex when you push down on them. I don’t think you’ll have any issues with them breaking after long-term use. The back also has rubber feet which will keep the Wacom One from sliding around your table.
The top of the tablet has a pen holder which is a nice touch and will keep you from losing it. There’s also one USB-C port on top which is used for the cable that connects to your computer and power adaptor. Aside from that, that’s pretty much with the Wacom One. It’s rather simple looking but gets the job done.
As for the Wacom One Pen, it’s pretty basic and not as good as the Intuos Pro Pen 2. It only has one button on the side and there is no eraser tip. It’s very comfortable to use, but it’s as basic as things get when it comes to stylus pens.
EXPERIENCE
While I enjoyed my time with the previous Wacom Intuos Pro, once you start using a pen tablet with a display on it, it’s pretty hard to go back to anything else. Although I was a bit annoyed by the way in which you have to run the cables for the Wacom One, that annoyance goes away once you have it all set up. I’m using it with a desktop PC so a lot of the wires get hidden anyways on the way to the PC. On the tabletop side, you only see one cable, so all-in-all not really a big deal.
As for actual usage, you’ll need to set up Wacom Center software to make full use of the Wacom One. It’ll probably download some drivers, maybe an update, and many of the bonus software you might want to use. I was a bit disappointed that much of the bonus software was only extended trials and not “keep forever-type” things. But I’m assuming that most of you who are going to be purchasing this probably already have some favorite software you already use.
Next up is the fact that the Wacom One not only functions as a pen tablet but also as a second display. You can either use it to extend your current work area or have it mirror your main display. I chose to extend the display because it allows you to work on a drawing for instance while also having another window up on a different display as a reference. Of course, the choice is yours how you choose to use it, but this is just how I use it.
Anyways, for the actual apps I used to test the Wacom One with, one of my favorites is Krita but I also tested this out with Wacom’s own Bamboo Paper app and also Photoshop.
All I can say is what an immediate improvement in accuracy when it comes to using the Wacom One. There really is nothing like being able to draw directly on a display and have that translate to a 1:1 thing with the media you’re working with. While working with a regular pen tablet is nice, there’s always a small amount of guesswork required when trying to do things. It’s still more accurate than a mouse when working with digital media but it’s not perfect. With a display on the tablet, it’s pretty much perfect. The Wacom One itself isn’t the best display tablet out there, but it’s still a great option for beginners.
IMPROVEMENTS
The design isn’t perfect and there are a few areas that could use improvement. For starters, the Wacom one does not have any shortcut buttons or dials. That means there’s no real quick way to manage the tools you use the most. You won’t be able to adjust tool size on the fly or swap to your most used digital tools. Instead, you’ll just have to use what’s on-screen like you’re using a mouse. The display also doesn’t support touch, so no gesture shortcuts either.
While the display is pretty nice, it could also be a bit brighter as I feel like it isn’t quite bright enough, even at its highest setting. I however really love the matte screen though which helps cut down glare and makes pen usage feel a lot more natural.
There are also a lot of cables you need to plug in and it won’t function wirelessly like the Intuos Pro line does. You however can’t really get around this because you need an HDMI connection for the display, so you’re going to have cables regardless.
FINAL THOUGHTS
The Wacom One isn’t meant to be a top-of-the-line item from Wacom. They have the Cintiq line for that. Instead, the Wacom One is, in my opinion, a starter pen display tablet for the masses. It has a lot of pretty good features and the build quality is actually quite good. While there are some things that could be improved or added, that doesn’t hinder the functionality of the Wacom One nor does it make it any less useful. It might not be a Pro-level display tablet, but for my needs, it’s perfect.
However, there is a lot of competition in the space right now so Wacom needs to stay on its toes if it doesn’t want to lose out here.
Thank You for Visiting TheGamerWithKids
I love bringing you guys the latest and greatest reviews whether it be apps or gadgets, but hosting and maintaining a website doesn’t come cheap. If you would like to purchase any of the items mentioned on the site, please do so by clicking our links to Amazon first and then purchasing the items. This way, we get a small portion of the sale and this helps me run the website. Thanks!
This review is made possible by my affiliation with G Style Magazine. These items were sent to us by the company or its PR reps for review and evaluation. It is also featured on the G Style Magazine website which is a tech blog that focuses on the fashion aspect of tech. Please visit their site for more interesting reviews on all your favorite gadgets and gear.
Wacom One review
The Wacom One is a great tablet for artists wanting to delve deeper into the digital drawing game.
Cons
Why you can trust Laptop Mag
Our expert reviewers spend hours testing and comparing products and services so you can choose the best for you. Find out more about how we test.
Drawing tablets such as the Wacom One cater toward seasoned artists, and their price points reflect that. The Wacom One breaks this cycle, offering artists a display tablet at a lower price, but with the reliability expected of a Wacom product. Plus, with the inclusion of Android support, it’s now easier to get into digital art for people who can’t afford a laptop to run painting software.
I remember how, years ago, the only option available for a display tablet was the Wacom Cintiq, which ran anywhere from 1,200 to 2,500. Competitors like Huion and XP-Pen have recently released much more affordable versions of these tablets, and as such, Wacom is taking strides to stay the “it” drawing tablet company. The Wacom One is the first budget-friendly display tablet Wacom has released, although at 399.95, some people would still consider that pricey.
The Wacom One is a great tablet for beginner or intermediate artists, or even hobbyists looking for the reliability of a Wacom product at a lower cost than some of the company’s more expensive alternatives.
Wacom One Price and Configuration Options
The only configuration of the Wacom One Pen Display is a 13.3-inch, 1080p model with a light-gray backing. There are no other color, size, or resolution options available. This tablet is currently available for 399.95 which, compared to the 799.95 Wacom Cintiq.- the only other display tablet Wacom makes.- is a much more reasonable price.
Wacom One Design
Surrounding the Wacom One’s 13.3-inch display are thick, black bezels which measure approximately a half inch on all sides. The screen is semi-matte, and uses plastic instead of glass in order to simulate the feel of paper. The only thing interrupting the solid light grey on the back of the Wacom One is a strip of black near the top, which is where you’ll find a kickstand that props the tablet up for comfortable drawing angles. Hiding under the kickstand legs are three replacement pen nibs, as well as a tool to help remove them from the pen.
One thing that stood out to me about the Wacom One was the lack of express keys. Normally, drawing tablets have anywhere between four and eight express keys, which can be programmed to shortcuts. Even the 69 Wacom Intuos CTL4100 has four programmable express keys. The XP-Pen 15.6” Pro display tablet has eight programmable express keys as well as a dial for actions such as zooming in and out, changing brush size, or scrolling up and down.
The lack of these features makes the experience of digital drawing more awkward, but it’s circumventable if you have a keyboard stationed nearby and the time to learn your drawing software’s shortcuts. The Wacom One can make your desk setup a little clunky even though the tablet is relatively small, measuring at only 14.1 x 8.9 x 0.6 inches.
Wacom One Ports
There isn’t much need for additional ports on drawing tablets, aside from a USB-C input, which is used to connect the Wacom One to the computer and to the power as well. The Wacom One comes with what they call an “X Cable”. The power plugs into the top of the tablet, and the cord is split into three separate cables. HDMI, USB-A, and a seperate USB to plug into the AC adapter. Yes, confusing, I know, and if you plug the wrong USB into the computer, the computer won’t recognize the tablet as a drawing device, which I discovered the hard way.
There isn’t even a place for the pen to plug into because Wacom pens, like the one included with this tablet, are cordless and battery-free.Instead, you get a flexible holder attached to the top of the tablet for storing the pen.
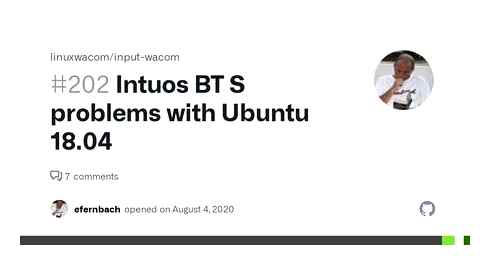
Wacom One Display
During the trailer for the movie Secret Garden, the Wacom One’s 13.3-inch, 1080p display performed decently. The greens were vibrant, but the purples seemed subdued. This is a little disappointing for an artist’s tablet, where you would hope that the colors and contrast would be accurate. According to our colorimeter, the Wacom One scored a 72% on the NTSC scale, which is about average for a tablet.
The screen is treated with an anti-glare film that makes drawing in a well lit area much easier because you aren’t constantly battling for the perfect angle to see what you’re drawing. While you can technically draw outside on a regular Android device, the sun tends to wash out colors a bit more, so it might be wise to keep the One indoors. Unfortunately I wasn’t able to measure the brightness for this tablet.
Wacom One Pen
Wacom once again blew me away with the natural feel of its pen. With 4,096 pressure levels, every line felt completely in control and accurate, just how it might come out on paper. Additionally, the tablet supports tilt angles up to 60 degrees, making paint brushes in Photoshop dynamic and easy to use. Unlike Wacom‘s normal pen design, the Wacom One’s stylus is much thinner and feels cheap.
This would normally be a downside, but the tablet is compatible with stylii produced by different companies, such as the Samsung Galaxy Note’s S Pen, Staedtler Noris Digital, and several more. This gives you the ability to personalize your drawing experience even further, though you’ll obviously be shelling out more cash if you opt for another company’s stylus.
I drew a few cool ladies in my time with the Wacom One, and it proved to be a very smooth drawing experience. Wacom has never let me down in this area. There was virtually no lag and no parallax which makes the experience as close to drawing on paper as you could want out of a tablet of this caliber. The pen has just one programmable button, which I programmed to pan/scroll to make moving around the canvas easier, but it’s possible to program any key, or combination of keys, to personalize your art process.
Wacom One Software and Warranty
In order to take advantage of the pressure-sensitive pen and the programmable button on said pen, you must download the Wacom One driver. Along with it comes the Wacom Desktop Center. This program works as a hub if you have multiple Wacom products. It’s an all-in-one program where you can customize settings, update drivers and request support.
The Wacom One also comes with a few other programs, such as Bamboo Paper, which is a cute program that simulates any kind of notebook you could imagine. With the Wacom One you get all of the Pro Pack options, which includes different brushes and book styles. It also comes with 2-month access to Adobe Creative Cloud, and a 3 month free trial of Clip Studio Paint Pro.
The Wacom One comes with a one-year warranty if you live in the US, Canada, Latin America, Asia Pacific or China, and a two-year warranty in Europe, Middle East and Africa.
Bottom Line
The Wacom One is catered toward the beginner artist who dreams of more. Wacom is no newcomer to drawing tablets, but it seems like it’s finally branching out to being more accessible to artists who aren’t beginners, but don’t quite have the income to drop 2,000 on a drawing tablet.
At 399.95, most people would agree that the Wacom One comes at a fair price. With 4,096 levels of pressure sensitivity and the natural, paper-like feel to the screen, drawing or writing comes almost as naturally as it would in a sketchbook or notepad. It would have been nice to see the inclusion of a few express keys in this otherwise well-rounded tablet.
If your goal is acquiring an on-the-go tablet, The Wacom One is not for you. Although it is lightweight, the tablet requires a power source at all times, and you need a USB-C hub if you wanted to connect it to your Android device. However, the Wacom One offers a very pleasant at-home or in-the-office experience.
If you’re looking to get into the digital drawing business and want the recognition and reliability of a brand name such as Wacom, the Wacom One is a great choice. If you’re looking for something a little more budget friendly, check out the XP Pen Artist12 Pro, although the drivers can be a bit spotty.
The new Wacom One drawing tablet is a great option for artists on a budget.
Our Verdict
The Wacom One is a solid entry into the budget drawing tablet market. For Windows and older Mac owners looking for an affordable drawing screen, the Wacom One should be high on the list. For newer Mac and Apple Pencil equipped iPad owners, the recent inclusion of SideCar makes it a harder sell.
For
- Excellent value for Windows users
- Authentic ‘Wacom’ drawing experience
- Well made out of premium plastics
Against
- No button on the screen unit
- Undesirable power lead position
- Screen brightness
Why you can trust Creative Bloq
Our expert reviewers spend hours testing and comparing products and services so you can choose the best for you. Find out more about how we test.
The new Wacom One is designed to be an affordable and, more importantly, an accessible new entry point for artists looking for a drawing tablet. The Wacom One is friendly both in its appearance and its ability to work with Windows, Mac and Android devices, most notably working with a variety of styluses other than the one it ships with.
Screen: 13 FHD Ports: Power Dimensions: 225 x 357 x 14.6 mm Weight: 1.0 kg
The Wacom One could be a step up for many new 2D and 3D artists looking to find more natural ways to create. At a fraction of the price of the Wacom‘s Cintiq displays, has a reduction in price meant a decrease in performance? Or is Wacom‘s latest release set to hit a spot in our round up of the best drawing tablets money can buy?
The Wacom One is the first drawing screens I’ve had access to for a long time. I used Wacom’s Cintiq 13 and Cintiq 21 approximately five years ago when they were needed for my visualisation work. However, both of these devices went to eBay heaven for a couple of reasons. Firstly because they always presented a cabling nightmare and secondly because they were big and cumbersome.
Also, the iPad Pro and the Apple Pencil happened. iPad applications like Duet and AstroPad mimicked the functionality of a Cintiq, and when the iPad was not being used as a drawing tablet or second screen, it could also be used as an iPad. So I was interested to see what the Wacom One could offer in a post-iPad world. Especially with the addition of SideCar, which allows a newer iPad to be used as the second screen or drawing display with more recent Macs with no additional applications.
Wacom One review: Design and accessories
When opening the packaging for the Wacom one, I was relieved to see that Wacom still puts effort into its packaging, everything I needed was accessible and available. However, there are still cables, this is both good and bad. Bad because who wants to mess with cables in this Wi-Fi world? The Wacom One needs three to connect to a computer; one for USB, one for HDMI and one for its own power, the latter of which is annoying. The good point about cables, however, is it means the device doesn’t draw power from a laptop, which is the case for iPad solutions.
On both Mac and Windows, the Wacom One was plug and play. Wacom haven’t skimped on dongles for the lower price. There are USB-C adaptors included for both the HDMI and USB cables, which meant that the Wacom One worked with no additional dongles needed for my two test computers (Razer Blade 15 and a 2017 15 MacBook Pro).
The Wacom One unit itself is housed in a very well made white utilitarian plastic case, that has a couple of fold-out legs which stow away flat when not in use. There is a single power-lead which has a HDMI and USB connector, and that’s it.
There is no adjustment in the legs, and the placement of the power lead means that it is difficult to turn the screen around so that it could sit as an upright second monitor, which feels like a missed opportunity.
The stylus that comes with the Wacom One is a more basic solution than the Pro styluses that I am used to with the Wacom Intuos range. In the little styluses defence, in use, as it has 4096 levels of pressure sensitivity, drawing felt as precise as it has always done with Wacom tablets. There is only one button on the stylus, which can be mapped to whatever the user desires using the Wacom drivers. I also missed a hardware button on the Wacom One itself.
Wacom One review: Screen
The screen resolution is 1920 by 1080, which for the size is absolutely fine, especially when working with Windows. Another size benefit is that viewing angles held up well for colour accuracy when viewed off centre, even though the screen can only manage 72% of NTSC colour gamut coverage.
The screen’s one major downfall is that it simply isn’t very bright, and this can be an issue in a room with overhead downlighting, as the matte surface spreads out diffuse reflections.
When compared with an iPad with an Apple Pencil, that can connect to Mac with the new SideCar software, the screen difference and quality is literally night and day in the iPads favour. SideCar also implements dynamic software buttons on the touch display of the iPad, making it, in my opinion, a better experience both in use and value. As when the artist is not using SideCar, they also have an iPad to use.
Wacom One review: Should you buy it?
The Wacom feel is ‘better’ than the iPad, in terms of latency. However, the drawing experience is identical on iPad or Wacom One, and a basic iPad and Pencil can be found for approximately the same price as a Wacom One.
For Window users, however it is a very different story. I have never been tempted by a drawing device other than Wacom, due to their reliability and feel. In this regard, the Wacom One maintains the Wacom brand well. The Wacom One is a robust and dependable device that always just ‘worked’ when I plugged it into the Razer laptop. Due to its flexibility, rugged construction and drawing experience, the Wacom One should be considered by any artist looking for their first drawing display – as long as they don’t work in room with lots of bright lights.